在标准C++没有提供专门用于套接字通信的类,所以只能使用操作系统提供的基于C的API函数,基于这些C的API函数我们也可以封装自己的C++类 C++套接字类的封装。但是Qt就不一样了,它是C++的一个框架并且里边提供了用于套接字通信的类(TCP、UDP)这样就使得我们的操作变得更加简单了(当然,在Qt中使用标准C的API进行套接字通信也是完全没有问题的
)。下面,给大家讲一下如果使用相关类的进行TCP通信。
使用Qt提供的类进行基于TCP的套接字通信需要用到两个类:
- QTcpServer:服务器类,用于监听客户端连接以及和客户端建立连接。
- QTcpSocket:通信的套接字类,客户端、服务器端都需要使用。
这两个套接字通信类都属于网络模块network
。
1. QTcpServer
QTcpServer
类用于监听客户端连接以及和客户端建立连接,在使用之前先介绍一下这个类提供的一些常用API函数:
1.1 公共成员函数
构造函数
1
| QTcpServer::QTcpServer(QObject *parent = Q_NULLPTR);
|
给监听的套接字设置监听
1 2 3 4 5 6 7
| bool QTcpServer::listen(const QHostAddress &address = QHostAddress::Any, quint16 port = 0);
bool QTcpServer::isListening() const;
QHostAddress QTcpServer::serverAddress() const;
quint16 QTcpServer::serverPort() const
|
- 参数:
- address:通过类
QHostAddress
可以封装IPv4
、IPv6
格式的IP地址,QHostAddress::Any
表示自动绑定
- port:如果指定为0表示随机绑定一个可用端口。
- 返回值:绑定成功返回true,失败返回false
得到和客户端建立连接之后用于通信的QTcpSocket
套接字对象,它是QTcpServer
的一个子对象,当QTcpServer
对象析构的时候会自动析构这个子对象,当然也可自己手动析构,建议用完之后自己手动析构这个通信的QTcpSocket
对象。
1
| QTcpSocket *QTcpServer::nextPendingConnection();
|
阻塞等待客户端发起的连接请求,不推荐在单线程程序中使用,建议使用非阻塞方式处理新连接,即使用信号 newConnection()
。
1
| bool QTcpServer::waitForNewConnection(int msec = 0, bool *timedOut = Q_NULLPTR);
|
- 参数:
- msec:指定阻塞的最大时长,单位为毫秒(ms)
- timeout:传出参数,如果操作超时timeout为true,没有超时timeout为false
1.2 信号
当接受新连接导致错误时,将发射如下信号。socketError参数描述了发生的错误相关的信息。
1
| [signal] void QTcpServer::acceptError(QAbstractSocket::SocketError socketError);
|
每次有新连接可用时都会发出 newConnection() 信号。
1
| [signal] void QTcpServer::newConnection();
|
2. QTcpSocket
QTcpSocket
是一个套接字通信类,不管是客户端还是服务器端都需要使用。在Qt中发送和接收数据也属于IO操作(网络IO),先来看一下这个类的继承关系:

2.1 公共成员函数
构造函数
1
| QTcpSocket::QTcpSocket(QObject *parent = Q_NULLPTR);
|
连接服务器,需要指定服务器端绑定的IP和端口信息。
1 2 3
| [virtual] void QAbstractSocket::connectToHost(const QString &hostName, quint16 port, OpenMode openMode = ReadWrite, NetworkLayerProtocol protocol = AnyIPProtocol);
[virtual] void QAbstractSocket::connectToHost(const QHostAddress &address, quint16 port, OpenMode openMode = ReadWrite);
|
在Qt中不管调用读操作函数接收数据,还是调用写函数发送数据,操作的对象都是本地的由Qt框架维护的一块内存。因此,调用了发送函数数据不一定会马上被发送到网络中,调用了接收函数也不是直接从网络中接收数据,关于底层的相关操作是不需要使用者来维护的。
接收数据
1 2 3 4 5 6
| qint64 QIODevice::read(char *data, qint64 maxSize);
QByteArray QIODevice::read(qint64 maxSize);
QByteArray QIODevice::readAll();
|
发送数据
1 2 3 4 5 6
| qint64 QIODevice::write(const char *data, qint64 maxSize);
qint64 QIODevice::write(const char *data);
qint64 QIODevice::write(const QByteArray &byteArray);
|
2.2 信号
在使用QTcpSocket
进行套接字通信的过程中,如果该类对象发射出readyRead()
信号,说明对端发送的数据达到了,之后就可以调用 read 函数
接收数据了。
1
| [signal] void QIODevice::readyRead();
|
调用connectToHost()
函数并成功建立连接之后发出connected()
信号。
1
| [signal] void QAbstractSocket::connected();
|
在套接字断开连接时发出disconnected()
信号。
1
| [signal] void QAbstractSocket::disconnected();
|
3. 通信流程
使用Qt提供的类进行套接字通信比使用标准C API进行网络通信要简单(因为在内部进行了封装)原始的TCP通信流程 Qt中的套接字通信流程如下:
3.1 服务器端
3.1.1 通信流程
- 创建套接字服务器
QTcpServer
对象
- 通过
QTcpServer
对象设置监听,即:QTcpServer::listen()
- 基于
QTcpServer::newConnection()
信号检测是否有新的客户端连接
- 如果有新的客户端连接调用
QTcpSocket *QTcpServer::nextPendingConnection()
得到通信的套接字对象
- 使用通信的套接字对象
QTcpSocket
和客户端进行通信
3.1.2 代码片段
服务器端的窗口界面如下图所示:
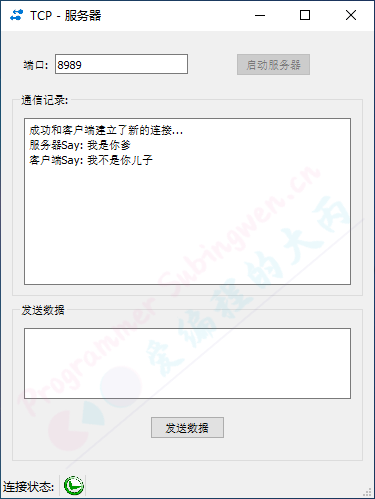
头文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| class MainWindow : public QMainWindow { Q_OBJECT
public: explicit MainWindow(QWidget *parent = 0); ~MainWindow();
private slots: void on_startServer_clicked();
void on_sendMsg_clicked();
private: Ui::MainWindow *ui; QTcpServer* m_server; QTcpSocket* m_tcp; };
|
源文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); setWindowTitle("TCP - 服务器"); m_server = new QTcpServer(this); connect(m_server, &QTcpServer::newConnection, this, [=]() { m_tcp = m_server->nextPendingConnection(); ui->record->append("成功和客户端建立了新的连接..."); m_status->setPixmap(QPixmap(":/connect.png").scaled(20, 20)); connect(m_tcp, &QTcpSocket::readyRead, this, [=]() { QString recvMsg = m_tcp->readAll(); ui->record->append("客户端Say: " + recvMsg); }); connect(m_tcp, &QTcpSocket::disconnected, this, [=]() { ui->record->append("客户端已经断开了连接..."); m_tcp->deleteLater(); m_status->setPixmap(QPixmap(":/disconnect.png").scaled(20, 20)); }); }); }
MainWindow::~MainWindow() { delete ui; }
void MainWindow::on_startServer_clicked() { unsigned short port = ui->port->text().toInt(); m_server->listen(QHostAddress::Any, port); ui->startServer->setEnabled(false); }
void MainWindow::on_sendMsg_clicked() { QString sendMsg = ui->msg->toPlainText(); m_tcp->write(sendMsg.toUtf8()); ui->record->append("服务器Say: " + sendMsg); ui->msg->clear(); }
|
3.2 客户端
3.2.1 通信流程
- 创建通信的套接字类
QTcpSocket
对象
- 使用服务器端绑定的IP和端口连接服务器
QAbstractSocket::connectToHost()
- 使用
QTcpSocket
对象和服务器进行通信
3.2.2 代码片段
客户端的窗口界面如下图所示:
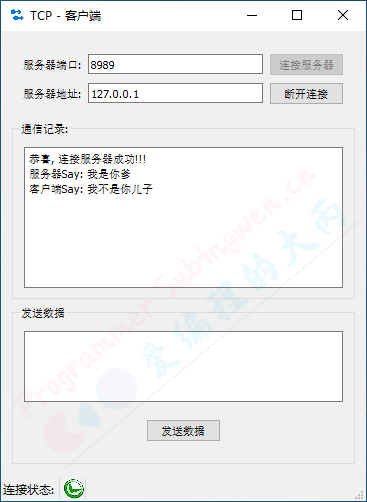
头文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| class MainWindow : public QMainWindow { Q_OBJECT
public: explicit MainWindow(QWidget *parent = 0); ~MainWindow();
private slots: void on_connectServer_clicked();
void on_sendMsg_clicked();
void on_disconnect_clicked();
private: Ui::MainWindow *ui; QTcpSocket* m_tcp; };
|
源文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| MainWindow::MainWindow(QWidget *parent) : QMainWindow(parent), ui(new Ui::MainWindow) { ui->setupUi(this); setWindowTitle("TCP - 客户端");
m_tcp = new QTcpSocket(this); connect(m_tcp, &QTcpSocket::readyRead, [=]() { QByteArray recvMsg = m_tcp->readAll(); ui->record->append("服务器Say: " + recvMsg); }); connect(m_tcp, &QTcpSocket::connected, this, [=]() { ui->record->append("恭喜, 连接服务器成功!!!"); m_status->setPixmap(QPixmap(":/connect.png").scaled(20, 20)); }); connect(m_tcp, &QTcpSocket::disconnected, this, [=]() { ui->record->append("服务器已经断开了连接, ..."); ui->connectServer->setEnabled(true); ui->disconnect->setEnabled(false); }); }
MainWindow::~MainWindow() { delete ui; }
void MainWindow::on_connectServer_clicked() { QString ip = ui->ip->text(); unsigned short port = ui->port->text().toInt(); m_tcp->connectToHost(QHostAddress(ip), port); ui->connectServer->setEnabled(false); ui->disconnect->setEnabled(true); }
void MainWindow::on_sendMsg_clicked() { QString sendMsg = ui->msg->toPlainText(); m_tcp->write(sendMsg.toUtf8()); ui->record->append("客户端Say: " + sendMsg); ui->msg->clear(); }
void MainWindow::on_disconnect_clicked() { m_tcp->close(); ui->connectServer->setEnabled(true); ui->disconnect->setEnabled(false); }
|